Accurately Calculate Your Electricity Cost Using a Jiansiyan Energy Module
by drmpf in Circuits > Arduino
104 Views, 0 Favorites, 0 Comments
Accurately Calculate Your Electricity Cost Using a Jiansiyan Energy Module
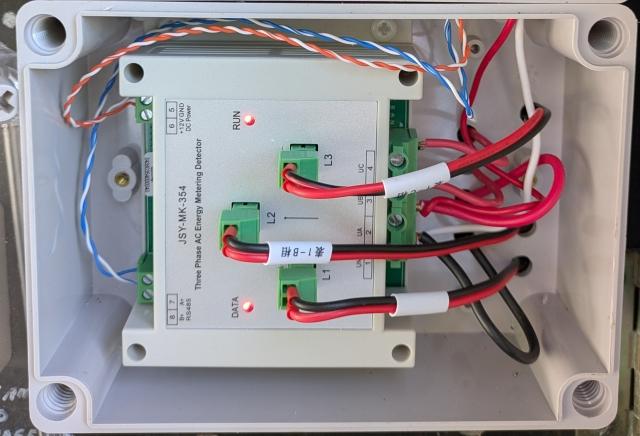
This Instructable shows how to use a Shenzhen Jiansiyan Technology Co. Ltd. AC bi-directional Electricity Energy Module to accurately calculate the electricity cost for your household. These modules are available in a wide range of types both single phase and 3-phase, with TTL-RS323 and RS458 connections. The modules provide instantaneous power measurements (250ms sampling interval) and separate values for positive (import) and negative (export) accumulated kWh measurements. One would expect that simply taking the difference between the accumulated positive kWh readings over a time period (and similarly for the negative kWh differences) would give you the number of kWh consumed from the electricity supply (or exported to back to the grid).
However the values calculated and stored in the JSY module are grossly inaccurate if the electricity flow switches from Import to Export.
As will be shown below, if the direction of the current flow (and hence the power) is ALWAYS in one direction, the JSY module's result is accurate. However if your house is fitted with has solar cells, or a battery, and exports your excess energy back to the mains, the JSY module's accumulated positive and negative kWh are completely un-useable.
To overcome this a simple code method is provided that integrates the instantaneous power and accumulates the Import and Export kWh. This method is shown to give result that agree with the electricity supply company's meter to within a few percent. The results from an Enphase Solar Cell monitor are also compared and found to be in close agreement with the both the electricity supply meter and the calculated values
Supplies
Jiansiyan Bidirectional Energy Meter Module - JSY-MK-354
2 x ESP32
Background

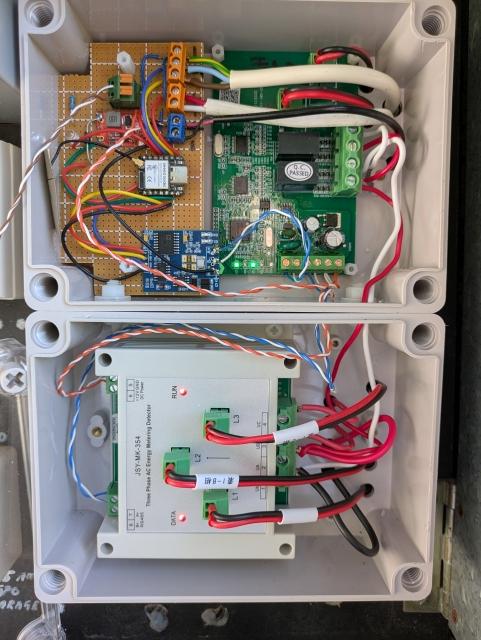
In August 2023 an IotWatt and a triac were installed to allow the hot water to be heated when there was excess solar power. This project also provided real time monitoring of the household power usage and daily cost.
In February 2025 the IotWatt was replaced with one based on JSY power monitors.
The new system was less expensive then the IoTWatt and controlled the hot water heating locally, without needing a stable WiFi network. Obtaining a reliable WiFi connection from inside the metal meter box can be problematic. While the JSY power monitors accurately measured the magnitude and direction of the instantaneous power and so could reliably turn the hot water heating element on and off as needed, it quickly became apparent that the import and export kWh accumulators in the JSY module where grossly in-accurate and that this was being reflected in the calculation of the daily electricity cost.
Checking the Accuracy of the JSY-MK-354 Energy Module


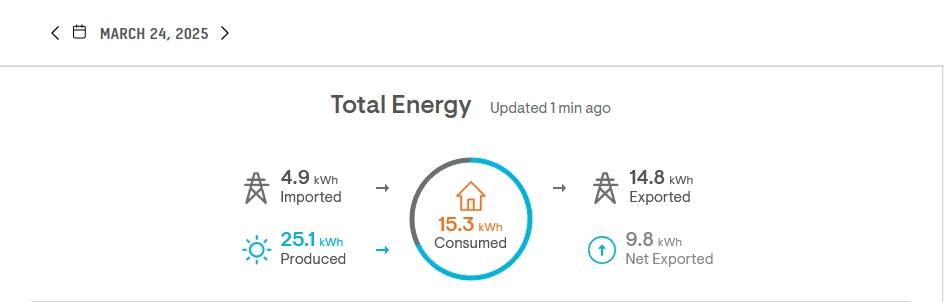
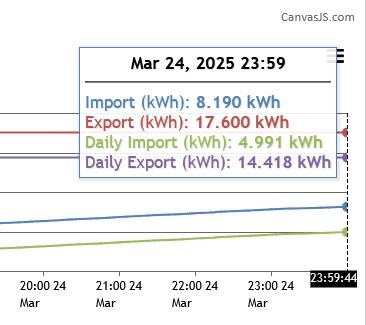
The specific JSY module used here was the 3-phase JSY-MK-354, if you are using another module you can use the procedure below to check its accuracy.
The JSY-MK-354 was mounted in the meter box together with an ESP32 micro-processor, which polled for the latest values every 2 seconds and forwarded them to a telnet connection. At the other end of the telnet connect was another ESP32 processor which timestamped the records and logged them to a daily file. (The detailed code and schematics for this installation will be given in a later instructable)
For the Import kWh, the JSY-MK-354 has a three-phase forward total active energy at address 013AH 013BH and for the Export kWh, a three-phase reverse total active energy at address 0142H 0143H, both of which are unsigned 16bit integers. Dividing them by 100 gives the kWh.
The primary check of the JSY module's accuracy is the electricity supply company's meter. On this house the meter has a 5G connection back to the electricity distributor, who then provides the imported kWh on a daily basis. The exported kWh are only reported monthly on the bill.
For 24th March 2025, the electricity supply company's meter reading was 4.915kWh. The dailyreading.csv contains the first and last measurement of the day from the day file, 20250324.csv
A simple subtraction of the JSY accumulating import_kWh readings gives 8.19kWh. That is the JSY module gives a measurement 60% higher then the supply meter reading.
Fortunately the household electricity cost is based on the electricity supply company's meter and NOT on JSY's measurement.
A second check of the JSY's accuracy is to compare its incremental import / export kWh against the measurements provided by the Enphase Solar Cell monitoring system. For 24th March 2025, the Enphase showed 4.9kWh imported and 14.8kWh exported. Note that the Enphase import value closely agrees with the electricity supply company's meter reading.
For the export kWh, the JSY delta accumulated export_kWh (584.97 – 567.37) is 17.5kWh compared to Enphase's measurement of 14.8kWh. Again the JSY module over states the export kWhr.
However when you check the net import-export you find, JSY's value is 8.19kWh – 17.6kWh = -9.4kWh. While Enphase net is 4.9kWh – 14.8kWh = -9.9kWh.
So the net import-export provided by the JSY module is reasonably accurate (within 5%), it is just the individual import and export kWhr that are grossly in error.
Unfortunately because the there is large difference between the price charged for imported power ($0.3674 / kWh) versus what is paid for exported power ($0.05 / kWh), the net import-export value is useless for calculating the daily electricity cost. You need accurate import and export kWh value and JSY's module does not supply these.
How to Calculate an 'accurate' Import/export KWh From the JSY Module
Having determined that the individual import and export kWhr values returned by the JSY module are grossly in-accurate leaves the problem how to calculate more accurate values.
The method used here to calculate the daily_export_kWh and daily_import_kWh (shown in the spreadsheet above) is to integrate the instantaneous real power (Watts) provide by the JSY module and add +ve results to the daily_import_kWh and -ve results (with the sign reversed) to the daily_export_kWh. As is shown in the spreadsheet above the daily_import_kWh integrated value is 4.992kWh which is within 1.5% of the supply meter's reading. The daily_export_kWh integrated value is 14.418kWh, which when compared to Enphase's measurement of 14.8kWh is within 2.5%.
Testing on other days gave similar results, that is the error between the calculated imported kWh and the electricity company's meter reading is less the +/- 1.5% While the JSY's values varied widely, +50% error was not uncommon.
Here is the code used to integrated the global variables dailyImport_kWh and dailyExport_kWh as each 2 secs, as power measurement comes in from the JSY module.
void integrate(float Power_W) {
static unsigned long lastTime_ms = 0;
unsigned long currentTime_ms = millis();
if (lastTime_ms > 0) { // Skip first reading
unsigned long deltaTime_ms = currentTime_ms - lastTime_ms;
if (deltatTime_ms < 6000) { // not a too large gap between measurements
float deltaTime_hrs = (float)deltaTime_ms / (3600.0 * 1000.0);
float power_kW = (float)Power_W / 1000.0; // power in kW
float energy_kWh = power_kW * deltaTime_hrs; // kWh for this time interval
// Update daily accumulators based on whether importing or exporting
if (power_kW >= 0) { // Importing
dailyImport_kWh += energy_kWh;
} else { // Exporting (power_kW is negative)
dailyExport_kWh -= energy_kWh; // Store as positive value
}
}
}
lastTime_ms = currentTime_ms;
}
This is a very basic integration, but as the results show, it is sufficient.
A sample of the file for one day is 20250324.csv. The export_kwh and import_kwh columns are the accumulated kWh values proved by the JSY-MK-354. The last two columns daily_export_kwh and daily_import_kwh are the 'accurate' daily accumulated export and import kWh calculated (as described above) to replace the in-accurate JSY values.
Why the JSY Energy Module's Accumulated KWh Is Inaccurate
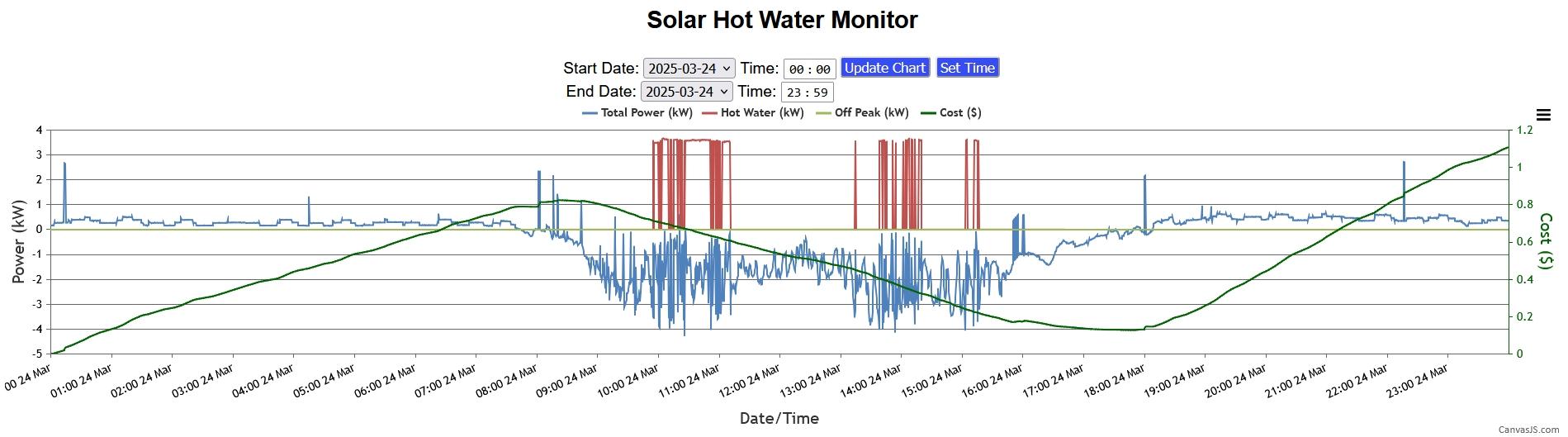

Using the data from 20250324.csv, the first plot is of the household instantaneous power (blue) and the hot water heater (red), and the cost(green).
The second plot compares JSY's Import/Export kWh to the calculated Daily Import/Export kWh
These plots show that there is little difference between JSY's values and the Daily calculated values as long as the power does not change direction. However once the hot water starts taking power, the household power changes from export to import a number of times. Each time the power changes to import, the hot water (the red plot in the first plot) is switched off. Then switched on again when there is >4kW being exported.
Compare the Red line, JSY export_kWh, versus the Purple line, Daily calculated export kWh, in the Energy Delta's plot. They start to diverge when the power starts to reverse at ~10am. After ~6pm they maintain a constant difference with no additional divergence as the power stays in one direction.
Since the JSY's net import-export value is correct, it appears that when the power flow changes, JSY adds some kWh to BOTH the accumulated Import and the accumulated Export kWh registers and this is the source of the inaccuracy in those readings.
Conclusion
This instructable covered how to get accurate Export / Import kWh measurements using a JSY-MK-345 Energy module. It checked the accuracy of the JSY-MK-345 against the electricity supply company's meter and found the JSY-MK-345 Export kWh value was 66% high over the day. Checking against the Enphase power monitor gave a similar result for the Import and found that the JSY-MK-345 Export kWh was some 20% high.
Having determined the JSY-MK-345 was un-useable for calculating the daily electricity cost, code for a much more accurate measurement of Import / Export kWhr was presented. This code performes a simple integration of the instantaneous power reported by the JSY-MK-345 module.
While the actual Export / Import kWh value accumulated by the JSY-MK-345 module were widely in-accurate, the net import-export kWh from the JSY-MK-345 module closely agreed with both the Enphase and the calculated (integrated) values. A plot of the divergence between the JSY-MK-345 values and the calculated (integrated) values showed that it was the change in instantaneous power direction that produced the errors in the JSY-MK-345.
Given these results, it would be prudent to undertake accuracy measurement on any Shenzhen Jiansiyan Technology Co. Ltd. energy module being used before relying on its results.