Approach Sensor With ESP32
by Fernando Koyanagi in Circuits > Microcontrollers
5815 Views, 7 Favorites, 0 Comments
Approach Sensor With ESP32
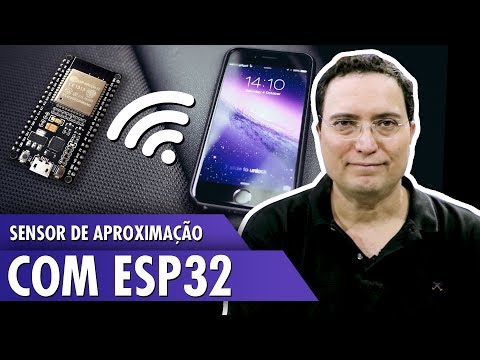
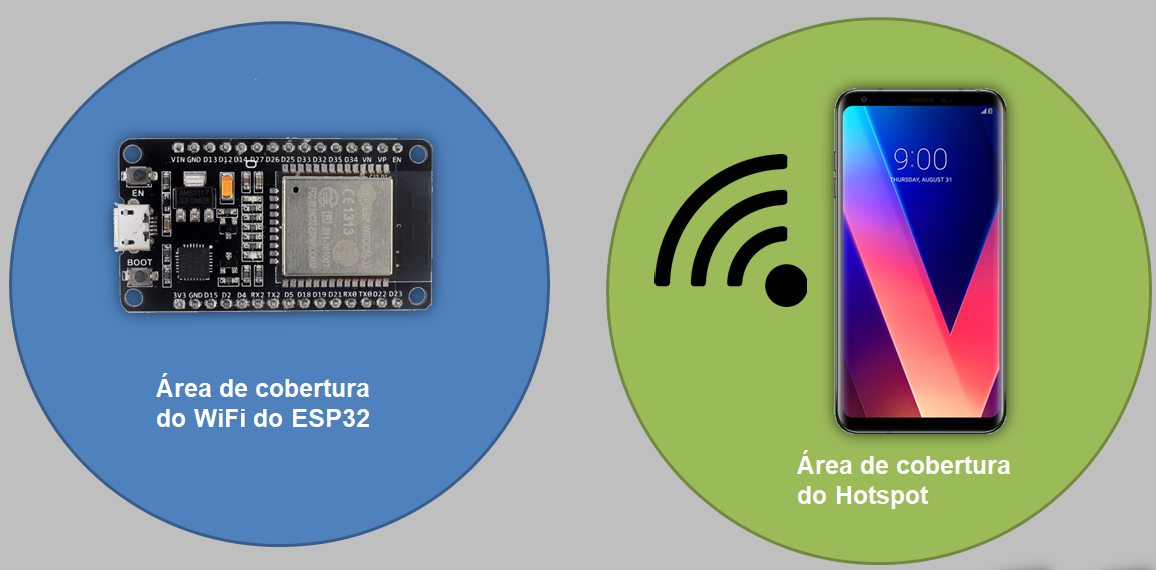
Would you like to leave your smart home using only an ESP32 and a smartphone? Would you like to arrive home and have the garage light already coming on, or the gate of your house open? What about having the air conditioning turn on when you approach a room? Do you like any of these ideas? This is all easily possible if we program a microcontroller and connect it to some sensors. And today, I'll show you how to do all this without using any sensors and no application to control. Check it out!
Well, the idea here is to program the ESP32 when the smartphone (which must be with the hotspot activated) arrives at a distance that allows the network to be recognized. Then, it will activate / deactivate some pin, which could be a relay (for example), which is the case today.
It is important to mention that nowadays, there are iTag and iBeacon that work through Bluetooth. However, our project is by RF. It’s also important to remember that there are advantages and disadvantages to using both Bluetooth and WiFi. Which is better? It is necessary to analyze case-by-case. The example I demonstrate here is quite simple, since my intention is to show you only the concept. But if you want to put in 50 position detectors (for example, let's say 50 ESPs), of course it will be necessary to improve the source code.
Demonstration

In our circuit, we have the ESP32 connected to our relay. I place the cell phone very close, and I see on the computer screen the value of RSSI that is being collected. The relay LED will illuminate when you are away from the phone.
WiFi NodeMCU-32S ESP-WROOM-32

Assembly

Program
Now let’s make a program in which the ESP32 will be scanning WiFi networks in its range. When you find the default network (which will be the smartphone hotspot), the pin that controls the relay will be activated. And the pin will be disabled when out of range.
Libraries and Variables
First, I will show you the options to compile libraries on both ESP32 and ESP8266. I define the name of the WiFi network that the microcontroller will fetch, and the term to be displayed if the relay is on or off. I also point out the pin that will control the relay and the minimum dB to identify the network.
#if defined(ESP8266)
#include <ESP8266WiFi.h> //ESP8266 Core WiFi Library #else #include <WiFi.h> //ESP32 Core WiFi Library #endif //nome da rede Wifi que o ESP irá buscar const char* SSID = “Meu_Hotspot”; #define RELAY_ON HIGH #define RELAY_OFF LOW //pino do rele #define RELAY 5 //dB minimo para identificar a rede #define MINdB -40
Setup
I initialize here the serial and the pin as output.
void setup() {
Serial.begin(115200); pinMode(RELAY, OUTPUT); // Inicializa o pino como saída. }
Loop
In the Loop, we look for the RSSI of the desired network. I check if the RSSI is higher than the desired minimum or if it is nonzero, which means that we have found the desired network. We then turn on the relay. Otherwise, we will disconnect.
void loop() {
int32_t rssi = getRSSI(SSID); //busca o RSSI da rede desejada // For debugging purpose only Serial.print("Signal strength: "); Serial.print(rssi); Serial.println("dBm"); //se o RSSI for maior que o mínimo desejado e o RSSI é diferente de zero (ou seja, encontramos a rede desejada) if (rssi > (MINdB) && rssi != 0) { digitalWrite(RELAY, RELAY_ON); //liga o relé Serial.println("ON"); } else { digitalWrite(RELAY, RELAY_OFF); //desliga o relé Serial.println("OFF"); } }
getRSSI
Here is the getRSSI function. It returns the RSSI of the fetched network (if it does not find it, it returns to zero). It scans the networks and compares if any of the ones found are what we want.
//retorna o RSSI da rede buscada (caso não ache, retorna zero)
int32_t getRSSI(const char* target_ssid) { byte available_networks = WiFi.scanNetworks(); //escaneia as redes for (int network = 0; network < available_networks; network++) { // Serial.print(WiFi.SSID(network)); Serial.print(" | "); Serial.println(WiFi.RSSI(network)); if (WiFi.SSID(network).compareTo(target_ssid) == 0) { //compara se alguma das redes encontradas é a que desejamos return WiFi.RSSI(network); //retorna o SSID da rede } } return 0; }