Arduino Esplora Mouse

Hello everyone, This is K'NEXnCoding bringing you another cool tutorial for you guys. This project lets you use your Arduino Esplora board to completely act like a mouse. It can move, left click, right click, and even scroll. The best part is that you don't even need to do anything.
However, it is a good idea to read to learn how to use this project, even though it is pretty straight forward.
How to Move the Mouse
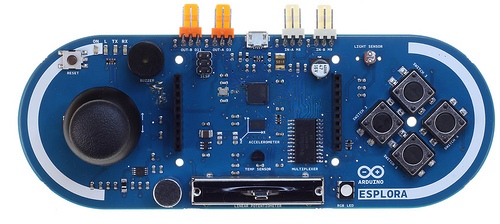
To move the mouse, just use the joystick. Move it up, down, left, right... You know, like normal. Also, you can press down on the joystick to left click.
Linear Potentiometer
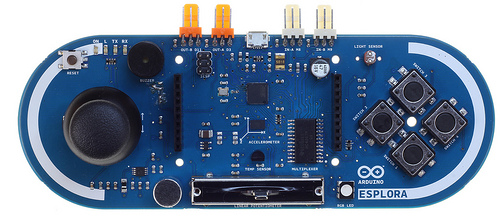
Use the linear potentiometer to control the sensitivity of the mouse movement.
Left and Right Click
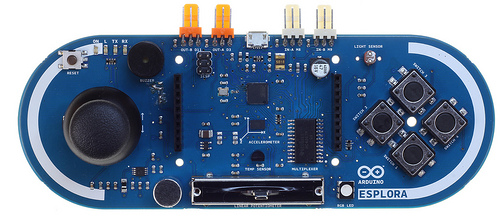
Again, straightforward. The left button left-clicks, and the right button right-clicks.
Scrolling
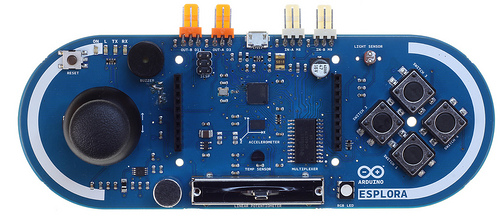
To scroll, use the top and bottom buttons.
The Code
Here is the code. Just copy and paste and your good to go!
Note: You can display the coordinates the mouse is at using the serial monitor, but sometimes it will cause Arduino to crash.
#include <Esplora.h> #include <Mouse.h>
void setup() { Mouse.begin(); }
int oldScrollUp = 0; int oldScrollDown = 0; int stateTime = 0;
#define THRESHOLD 20
void loop() { int xValue = Esplora.readJoystickX(); int yValue = Esplora.readJoystickY(); Serial.print("Joystick X: "); // print a label for the X value Serial.print(xValue); // print the X value Serial.print("\tY: "); // print a tab character and a label for the Y value Serial.print(yValue); // print the Y value int leftButton = Esplora.readJoystickSwitch() && Esplora.readButton(SWITCH_LEFT); int rightButton = Esplora.readButton(SWITCH_RIGHT); int scrollUp = Esplora.readButton(SWITCH_UP); int scrollDown = Esplora.readButton(SWITCH_DOWN); int range = Esplora.readSlider(); int mouseRange = map(range, 0, 1023, 2, 10); int mouseX = (xValue < THRESHOLD && xValue > -THRESHOLD) ? 0 : map(xValue, -512, 512, mouseRange, -mouseRange); int mouseY = (yValue < THRESHOLD && yValue > -THRESHOLD) ? 0 : map(yValue, -512, 512, -mouseRange, mouseRange); int wheel = 0; if (oldScrollUp != scrollUp || oldScrollDown != scrollDown) { wheel = (scrollUp != scrollDown) ? (scrollUp ? -mouseRange : mouseRange) : 0; oldScrollUp = scrollUp; oldScrollDown = scrollDown; stateTime = millis(); } else if ((millis() - stateTime) % 100 == 0 && (millis() - stateTime) >= 1000) { wheel = (scrollUp != scrollDown) ? (scrollUp ? -mouseRange : mouseRange) : 0; } Mouse.move(mouseX, mouseY, wheel); if (!leftButton) Mouse.press(MOUSE_LEFT); else Mouse.release(MOUSE_LEFT); if (!rightButton) Mouse.press(MOUSE_RIGHT); else Mouse.release(MOUSE_RIGHT); }
Let me know if you tried it! Comment below.