Arduino Motion Sensing Alarm


This instructable will show how to make a functioning motion sensing alarm with an arduino uno.
Supplies

The materials needed for this are:
1 Arduino Uno
1 PIR Motion Sensor
1 LED
1 Piezo Buzzer
1 Breadboard
1 Jumper Wires
A computer
Wire the Pir Sensor
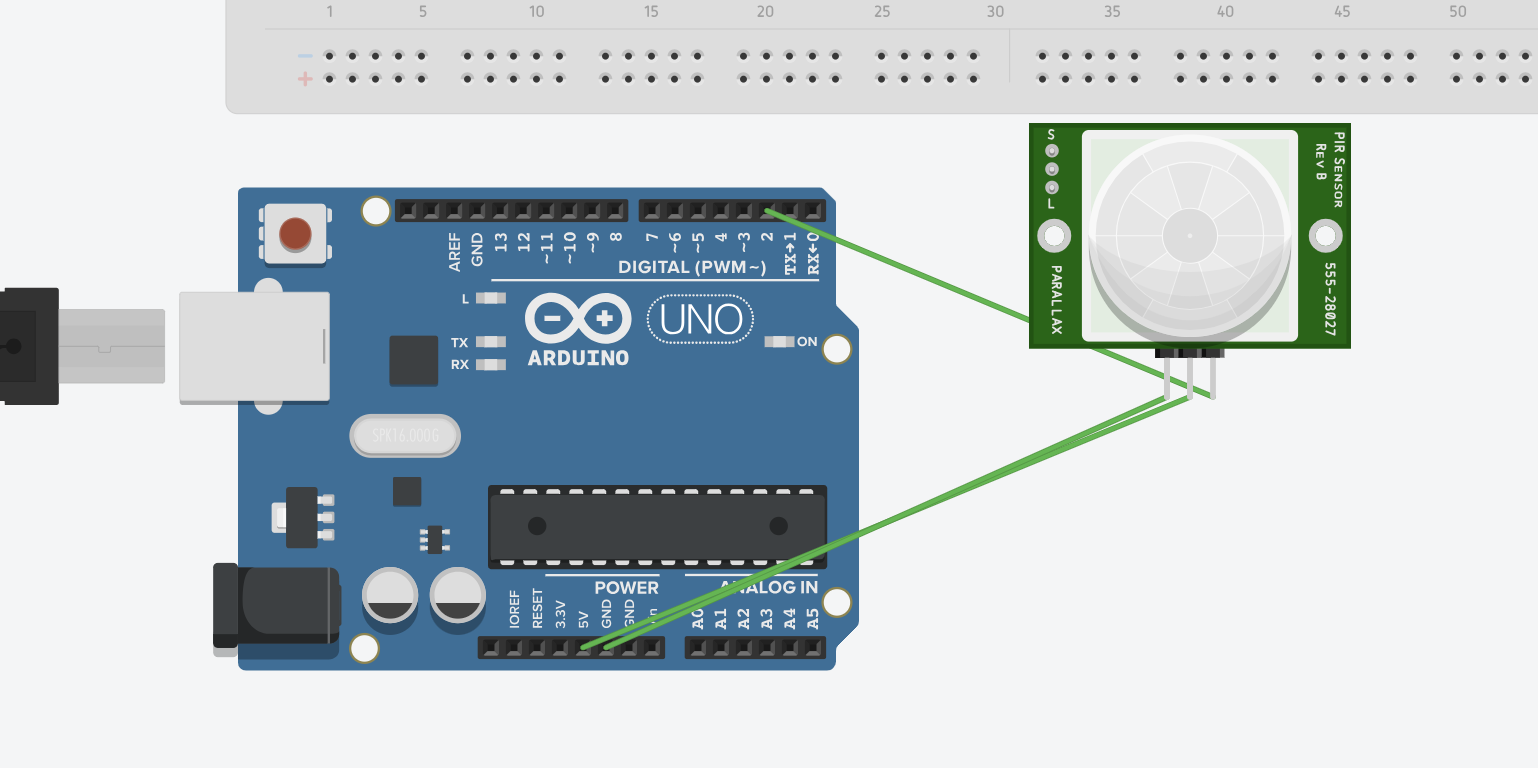
The pir sensor has 3 pins
The first on the left must be connected to a GND pin
The one in the middle must be connected to a digital pin(2)
The one on the right must be connected to a 5v pin
Wire the Piezo Buzzer
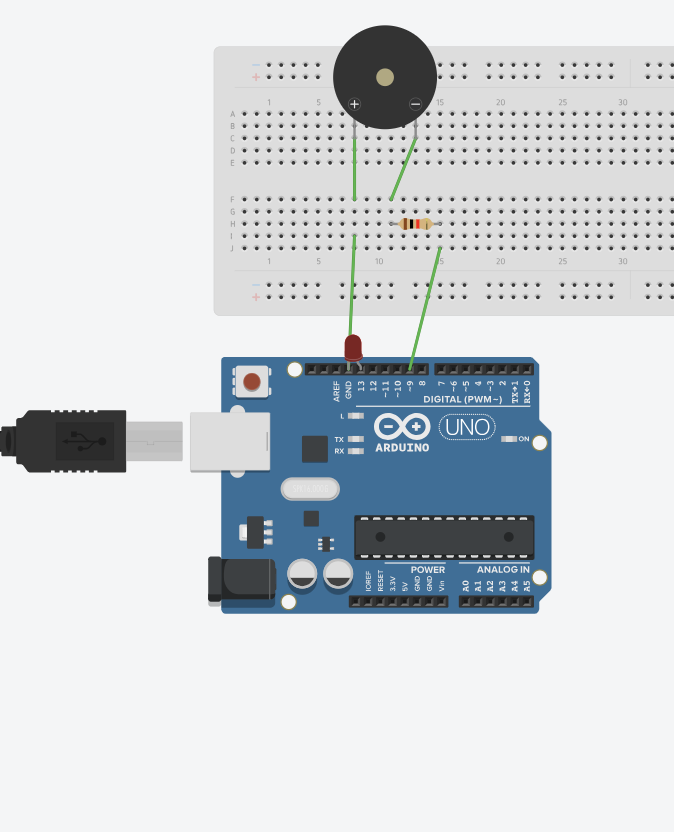
The piezo buzzer has 2 pins
One has to connect to a GND
The other has to connect to a digital pin
Wire Led

The LED has two pins, the ANODE and the CATHODE
The Anode is longer and is always wired to positive voltage
The Cathode is shorter and always wired to negative voltage
Wire the Anode to pin 13
Wire the Cathode to the GND pin next to it
Code for the Arduino
int ledPin = 13;
int inputPin = 2;
int pirState = LOW;
int val = 0;
int pinSpeaker = 10;
void setup() {
pinMode(ledPin, OUTPUT);
pinMode(inputPin, INPUT);
pinMode(pinSpeaker, OUTPUT);
Serial.begin(9600);
}
void loop(){
val = digitalRead(inputPin);
if (val == HIGH) {
digitalWrite(ledPin, HIGH);
playTone(300, 160);
delay(150);
if (pirState == LOW) {
Serial.println("Motion detected!");
pirState = HIGH;
}
} else {
digitalWrite(ledPin, LOW);
playTone(0, 0);
delay(300);
if (pirState == HIGH){
Serial.println("Motion ended!");
pirState = LOW;
}
}
}
void playTone(long duration, int freq) {
duration *= 1000;
int period = (1.0 / freq) * 1000000;
long elapsed_time = 0;
while (elapsed_time < duration) {
digitalWrite(pinSpeaker,HIGH);
delayMicroseconds(period / 2);
digitalWrite(pinSpeaker, LOW);
delayMicroseconds(period / 2);
elapsed_time += (period);
}
}
Reflection
During making this instructable I struggled a lot because I couldn't even make the arduino. After days of working on both the code and the wiring I finally got it correct and learned a lot a bout debugging codes. I learned where I have to put indents and how they make the code functions and with my partner helping out with the wiring it started becoming easier over time. Now I know a lot of new aspects of coding that I did not before and making arduinos has become easier.