Availability Indicator

Since i work at my home office, my wife asked me a way to quickly know if i'm available or not.
So i built a small availability indicator, controlled remotly by Bluetooth.
For this, i used :
- Teensy v2
- a HC-05 (Linvor) bluetooth module
- a servo motor
- some wires
- a piece of wood
- paper, scissor, glue, tape etc...
Hardware


It's quite simple, just connect as shown.
I used a Teensy without header pins, so the wires are directly soldered on the board
Frame


The servo is blocked by nails, on the top of the wooden piece.
Use the attached pdf file, print it, and glue it on a piece of cardboard, then cut . On the top the board, a hole was digged to allow the servo axis.
Same for the arrow (print, glue, cut), and it was fixed on the servo arm with tape.
Software
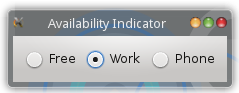
There is two pieces of code : one for the Teensy, and one for the computer
Teensy
The Teensy code is Arduino compatible. We manage the Bluetooth module with the HardwareSerial library
#include
#define MOTOR_PIN 4
HardwareSerial bt = HardwareSerial();
Servo myservo;
char c;
int pos = 0;
char intBuffer[12];
String intData = "";
int delimiter = (int) '\n';
void setup()
{
Serial.begin(9600);
myservo.attach(MOTOR_PIN); // attaches the servo on pin 9 to the servo object
bt.begin(9600);
bt.println("Start !!");
myservo.write(130); // Force 'Free' position at startup
}
void loop()
{
while (bt.available() ) {
delay(3);
int ch = bt.read();
if (ch == -1) {
// Handle error
Serial.println("No Data");
}
else if (ch == delimiter) {
Serial.println("delimiter -> break");
break;
}
else {
intData += (char) ch;
}
}
if (intData.length() > 0) {
// Copy read data into a char array for use by atoi
// Include room for the null terminator
int intLength = intData.length() + 1;
intData.toCharArray(intBuffer, intLength);
// Reinitialize intData for use next time around the loop
intData = "";
// Convert ASCII-encoded integer to an int
int pos = atoi(intBuffer);
if(( pos > 0 ) && (pos <= 200 ) ) {
//Serial.print ("go to pos : ");
//Serial.println(int(pos) );
myservo.write(pos);
pos = 200;
}
}
}
Desktop
I sent the servo position manually from the python code. Mine was 130 for 'Free' position, 70 for 'Work', and '25' for 'Phone'. You can calibrate it using a serial app (like cutecom).
You must insert your Bluetooth module MAC adress. You can obtain it with hcitool command
user@laptop:~ > hcitool scan
Scanning ...
00:xx:xx:xx:12:80 HC-05
user@laptop:~ >
As we use RFCOMM, we need to setup the linux box
user@laptop:~ > cat /etc/bluetooth/rfcomm.conf
#
# RFCOMM configuration file.
#
rfcomm0 {
bind yes;
device 00:13:03:19:12:80;
channel 1;
comment "BT-001";
}
user@laptop:~ >
user@laptop:~ > cat /var/lib/bluetooth/<your_BT_adapter_addr>/pincodes
00:13:03:19:12:80 1234
user@laptop:~ >
Downloads
Final
The indicator is action !