Interesting Programming Guidance for Designer--Program Process Control--Condition Statement (Part Two)
by ElecFreaks in Circuits > Electronics
110 Views, 0 Favorites, 0 Comments
Interesting Programming Guidance for Designer--Program Process Control--Condition Statement (Part Two)
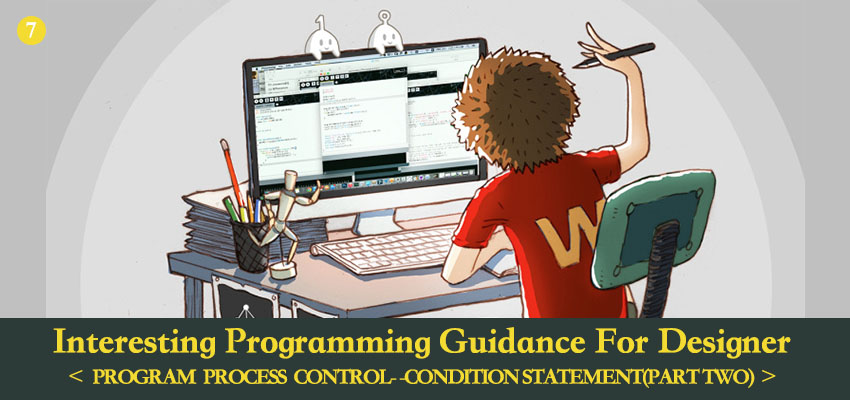
The final goal of this chapter is to complete a text venture game. So we have to know how to display texts and pictures in our program. The previous text outputs are all from the console. However, when we exported the program, we did not actually see the console. This requires us to think out of an idea to display texts in the window.
Simple Ways to Display Text
If you have no requirements towards font, then you can realize the display effect with only one command.
Code Example (7-1):
[cceN_cpp theme="dawn"] void setup(){
size(400,400);
}
void draw(){
background(0);
text("Font is here",50,50);
text("字体在这里",50,150);
} [/cceN_cpp]
Invoke Format:
text(str,x,y);
The usage of function text is to draw font in the window. The parameter str is for text content. It often adopts string type. So we have to add a double brace to declare the variable type. Besides, it can write datas like integer type, floating point type, etc..
Parameter x, y stand for the horizontal and vertical coordinate of text display.This coordinate will decide the position of the character's left bottom point.
We can directly input English characters. But when you try to input Chinese characters into function text, you will find that you can not directly input Chinese into Processing. The only way you can do is to copy and paste. Such as prepare the chinese in txt file , and then copy and paste it into Processing.
Sometimes the copied chinese characters will not be displayed properly. At this time, you can choose from the toolbar for Processing - Preference.
Choose from font column for a font that supports chinese character display such as Andale Mono. Meanwhile click to choose Enable complex text input , then it will display properly.
Use text command directly and then font will be set to the defaulted size. If you want to display some variables in the window instead of at the console, you can choose this method to save your time.
Set Font Size
Code Example (7-1-1):
[cceN_cpp theme="dawn"] void setup(){
size(400,400);
}
void draw(){
background(0);
textSize(50);
text("Font is here",50,50);
text("字体在这里",50,150);
} [/cceN_cpp]
Function textSize can be used to set the font size. But it is only suitable for English fonts. You have to use another method to set Chinese fonts.
Display Chinese Characters
Chinese character display in Processing is a little bit complicated. But once we figured it out, we will feel more covenient in latter use. It builds its fonts mainky through createFont command.
Code Example (7-2):
[cceN_cpp theme="dawn"] PFont font;
void setup(){
size(700,700);
font = createFont("SourceHanSansCN-Medium",30);
}
void draw(){
background(0);
textFont(font, 100);
text("中文字体", mouseX,mouseY);
} [/cceN_cpp]
Code Explain:
The first sentence desclare a PFont type. It is similar to int, float. But it stores fonts instead of numbers. The font behind represents variable name. We can name it randomly.
A PFont type built means we have a container. We have to tell the program what will be placed into the container. Function createFont () in setup is for uploading a font called "SourceHanSansCN-Medium" to font. This is a chinese font. The second parameter will decide the size of font. However, after real practice, this parameter do not have much influence and it will be replaced by other functions behind. So here we can write 30 firstly.
Some of you might wonder how to find the font called "SourceHanSansCN-Medium" and how to find the name of the font that we want to use.
You can move your mouse upon function createFont, click the right mouse key and choose Find in Reference to read instructions in its official website document. (If you want to know the usage of a certain function in detail, this can be a good option for you. )
In the above, we have refferred a method that we can output all fonts in the console by increasing two commands only.
Latter, you only have to copy the relative fonts and paste it into createFont command. Till this step, if you still not clear about the relative fonts of these English charasters, then you can search in the font library.
Back to the code. Function textFont in draw is used for setting. It stands for using the loaded font within variable font to display. While the second parameter is for the size of font. It is based on pixel unit here.
Later on, if we use function text again, it can display chinese characters normally.
Change Font Color
We can regard fonts as graphics. It is just like ellipse or rect that we can control font color with fill.
Code Example (7-3):
[cceN_cpp theme="dawn"] PFont font;
void setup() {
size(700, 700);
font = createFont("SourceHanSansCN-Medium", 30);
}
void draw() {
background(255);
textFont(font, 100);
fill(125);
text("中文字体", 150, 300);
fill(255, 155, 100);
text("English", 150, 500);
} [/cceN_cpp]
Display Pictures in the Window
Similar to create fonts, there are data types mainly for pictures. Before loading pictures into our program, we have to place pictures into a relative file.
Choose from the menu bar for "Sketch", and click "Show sketch folder", then you can put your pictures directly into it. It is in the same class with pde source file.
After we finished our preparation, we can start to write code.
Code Example (7-4):
[cceN_cpp theme="dawn"] PImage pic;
void setup(){
size(700,700);
pic = loadImage("test.jpg");
}
void draw(){
background(200);
imageMode(CENTER);
image(pic,width/2,height/2,400,400);
} [/cceN_cpp]
Code Explain:
PImage a kind of data type used for storing pictures.
The function of loadImage is to upload "test.jpg" pictures.
The usage of imageMode(CENTER) is to set the display mode of picture. If we do not add it, then the picture drawing method and the square defaulted drawing method will be the same. Its coordinate is the end point id the left top corner. Once we start this command, the coordinate will center on picture.
The usage of function image() is to display pictures. It has multiple parameter input modes. You can add 3 parameters or 5 parameters. When the parameter quantity is 3, the first parameter shall be variable PImage, the second and third parameter will decide the horizontal and verticle coordinate of the picture. At this time, the picture drawn to the window will coordinate with the size of source picture. When parameter quantity is 5, the former 3 parameters are completely same with when parameter quantity is 3 but the latter 2 parameters can decide the length and width of the picture.
Set Picture Color
Code Example (7-5):
[cceN_cpp theme="dawn"] PImage pic;
void setup(){
size(700,700);
background(255);
pic = loadImage("dog.png");
}
void draw(){
imageMode(CENTER);
tint(mouseX/(float)width * 255,255,255);
image(pic,mouseX,mouseY,200,200);
} [/cceN_cpp]
Code Explain:
Here, the loaded picture format is png, which has transparent background. Therefore, the graphic drawn by it will not have obvious square frame.
tint can be used to set the color of picture. It determines the proportion of light in each channel of the picture. Suppose the color value of a pixel is (r,g, b, a). After applying tint(A,B,C,D), the color of the new pixel becomes to (r (A/255),g (B/255),b (C/255),a (D/255)). tint is a sort of like a filter, which will execute the same operation to all pixels in the picture. When you do not write tint, the program defaults to run tint (255). This is to fully recover the oringinal color of the picture.
In the example, we use mouseX to change the first parameter of tint, which enable to make the release amount of the red channel grow from 0% to 100%. When we move the mouse to the left, the red light will decrease, while the green and blue light will appear. So we can see the profile image of doggy will appear to have green-blue color.
Comprehensive Application: Text Venture Game
I hope you have already laid a good foundation for the previous taught knowledges before developing games. Now you have owned all conditions to make a text venture game.
What is text venture game? The popular lifeLine not long ago can be classified into this group. If you are a PSP master, you might have heard Ace Attorney series. They are excellent artworks among text venture games.
If statement provides the possibility of building program bone frame. Now you need tofind "flesh" for it and attach them onto the bone frame. If you wish the world you built has more senses of substitution, the picture materials are necessary. So please prepare your relative picture materials before coding.
Even if you can not paint nor design, this do not represent you can't make games. Not only for the code, but also there are many game picture resources are opened online. These resources are allowed to be used to personal artworks or commercial artworks. For example: http://opengameart.org/ . The picture materials in the program below all come from this website.
Links of Game Picture Resources:
http://opengameart.org/content/roguelike-bosses (Boss)
http://opengameart.org/content/forest-background (Background )
http://opengameart.org/content/gold-treasure-icons... (Gold)
http://opengameart.org/content/animated-skeleton (Skeleton)
http://opengameart.org/content/pink-potion-item (Drug Bottle)
Font: SourceHanSansCN-Medium, an open font created by Adobe and Google.
Let's take a look of the final effect of our example first:
It looks so real! But the code below do not surpass the previous taught knowleges.
Code Example (7-6):
[cceN_cpp theme="dawn"]
PFont font; int count; PImage background, bottle, boss, bone, gold;void setup() { size(700, 400); font = createFont("SourceHanSansCN-Medium", 50);
bottle = loadImage("bottle.png"); background = loadImage("background.png"); boss = loadImage("boss.png"); bone = loadImage("bone.png"); gold = loadImage("gold.png");
count = 0; }
void draw() { background(0); textFont(font, 50); textSize(20);
//Draw Back Ground fill(255); imageMode(CENTER); image(background, width/2, height/2, 700, 400);
//Draw semi-transparent background behind texts. fill(0, 150); rect(0, 250, 700, 80); rect(0, 350, 700, 40);
if (count == 0) { fill(255); text("To find the legendary treausre, you entered a dark forest.", 30, 280); text("Suddenly, a mysterious bottle appeared. What will you do? ", 30, 310); fill(150, 150, 255); text("1. Kick it away. ", 30, 380); text("2. You drink it.", 180, 380); text("3. Your friends drink it.", 330, 380);
fill(255); image(bottle, width/2, height/3, 100, 100); }
if (count == 1) { fill(255); text("Suddenly the bottle turned over and a thick smoke rised. ", 30, 280); text("The final Boss appeared in front of you. You are going to ", 30, 310); fill(150, 150, 255); text("1. Run! ", 30, 380); text("2. Fight! ", 240, 380); text("3. Chat with it.", 500, 380);
fill(255); image(boss, width/2, height/3, 100, 100); }
if (count == 2) { fill(255); text("Suddenly, you owned super power. ", 30, 280); text("You heard the calling of the treasure and come to the final boss. You are going to...", 30, 310); fill(150, 150, 255); text("1. Fight directly. ", 30, 380); text("2. Chat with it. ", 250, 380);
fill(255); image(boss, width/2, height/3, 100, 100); }
if (count == 3) { fill(255); text(" You friends kneel down to the groud miserably. ", 30, 280); text(" Change to the final Boss in front of you. You are going to ", 30, 310); fill(150, 150, 255); text("1. Run ! ", 30, 380); text("2. Fight ! ", 240, 380); text("3. Chat with it. ", 500, 380);
fill(255); image(boss, width/2, height/3, 100, 100); }
if (count == 4) { fill(255, 0, 0); imageMode(CENTER); image(background, width/2, height/2, 700, 400); fill(255); text(" You are too slow. Boss casted a fire ball to you. ", 30, 280); text(" You can not fend due to big ability difference.", 30, 310); fill(150, 150, 255); text("Game Over", 30, 380);
fill(255); image(bone, width/2, height/3, 100, 100); }
if (count == 5) { fill(255); text("Boss is beaten. ", 30, 280); text("The treasure appeared.", 30, 310); fill(150, 150, 255); text("Take the treasure and run away from the dark forest. Succeed!", 30, 380);
fill(255); image(gold, width/2, height/3, 170, 150); }
if (count == 6) { fill(255, 0, 0); imageMode(CENTER); image(background, width/2, height/2, 700, 400); fill(255); text("Boss rejected your requirement and casted a fire ball to you. ", 30, 280); text(" You can not fend due to big ability difference.", 30, 310); fill(150, 150, 255); text("Game Over.", 30, 380);
fill(255); image(bone, width/2, height/3, 100, 100); } }
void keyPressed() { if (count == 0) { if (key == '1') { count = 1; } if (key == '2') { count = 2; } if (key == '3') { count = 3; } } else if (count == 1 || count == 3) { if (key == '1') { count = 4; } if (key == '2') { count = 5; } if (key == '3') { count = 6; } } else if (count == 2) { if (key == '1') { count = 5; } if (key == '2') { count = 6; } } } [/cceN_cpp]
Note: You can download the game code and resources here.
Code Analysis:
In order to present the process, the above example has designed two selection times only.
The example is for displaying the basic usage only. There are more spaces for you to explore and supplement in this area.
The variable count among it stands for a counter. We can use it to mark different backgrounds. Press different keys, you can switch among different backgrounds. Also, function draw will change with count to create the relative background.
Think
Can we use string to simplify our code?
Let's try more complicated branch structions, or even to animate the roles and endow them with life and force so that it can fight with the final big Boss.
Configure the Battle Animation to the Background
Export Your Artwork
After you finished a similar game, you might hope to share it with others immediately. To export your program from Processing is very easy. You just have to choose from the menu bar for File and Export Application.
Then click to choose export platform so that it will generate a file that can be executed. Copy this file to others. Just like the normal program, it can operate once the file opened. And other users can properly use without installing Processing.
End
I hope this chapter can stimulate your creation desire. There are more funny things in the program world waiting for you to explore.
This article comes from designer Wenzy.
Relative Readings:
Interesting Programming Guidance for Designer——Processing Initial Touch
Interesting Programming Guidance for Designer——Create Your First Processing Program
Interesting Programming Guidance for Designer–Get Your Picture Running(Part One)
Interesting Programming Guidance for Designer–Get Your Picture Running(Part Two)
Interesting Programing Guidance for Designer–Program Process Control- Loop Statement
Interesting Programming Guidance for Designer–Program Process Control–Condition Statement (Part One)
Source
This article is from: https://www.elecfreaks.com/11256.html
If you have any questions, you can contact: louise@elecfreaks.com.