Mesmerizing Chinese Dragon

Chinese culture has always fascinated me, from the precise symbols of the language to the way their cities and buildings flow seamlessly along. The idea of a "chinese dragon" brings to mind a snakelike, red and yellow creature, flying peacefully through the sky. I wanted to try and create this mental scene in a mesmerizing animation. To give myself a bit of a challenge, I decided to do this in Javascript, a programming language not particularly well suited to animation. So let's buckle up and start animating!
Supplies
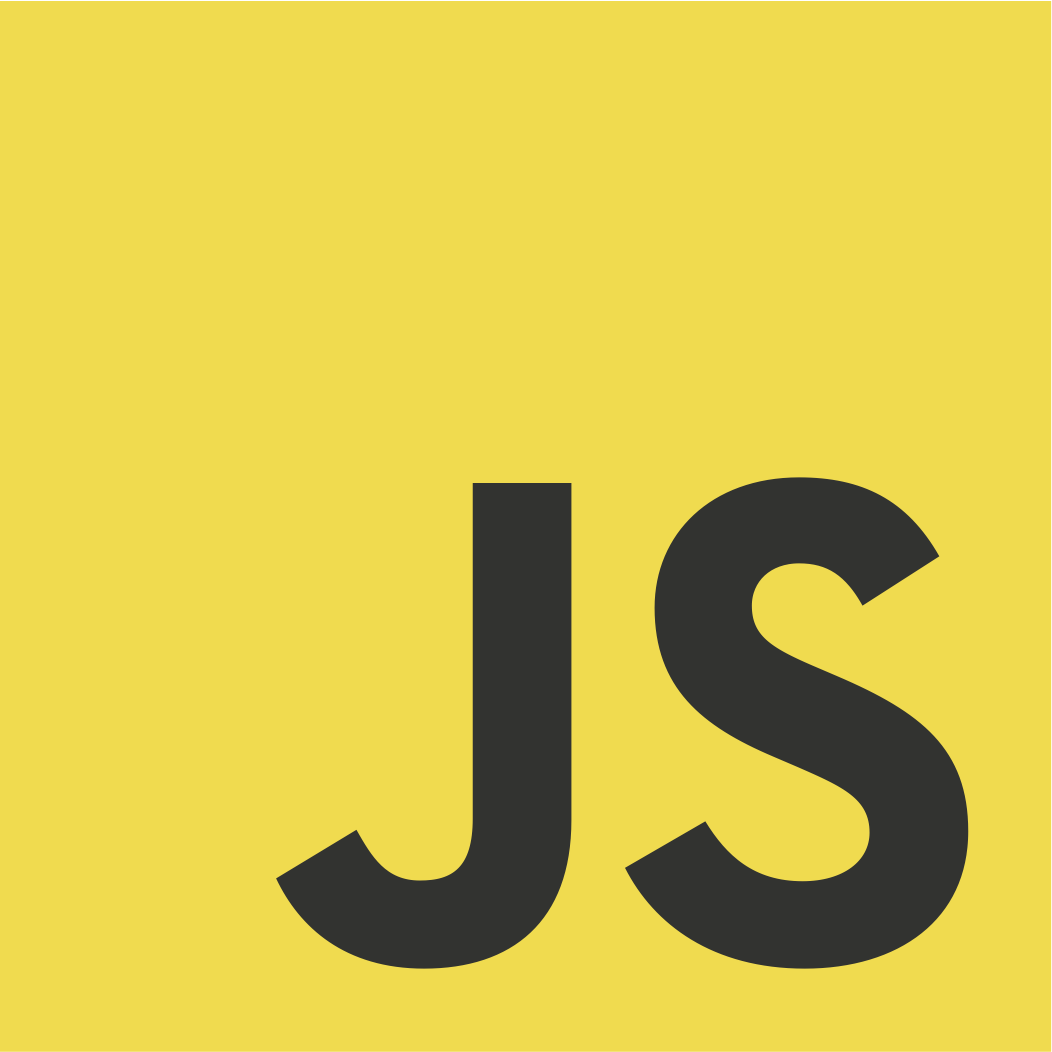
All you need for this project is a computer! Javascript runs in your browser, so you won't need to set anything up for the language itself. A basic understanding of the language is useful, but not strictly necessary. You can just copy-paste the code if you need, although I would encourage you to try and learn from it.
I'm going to be covering the idea behind the code first, which you can try and recreate yourself if you know Javascript, but if not, the last section has the code files themselves.
Skeleton
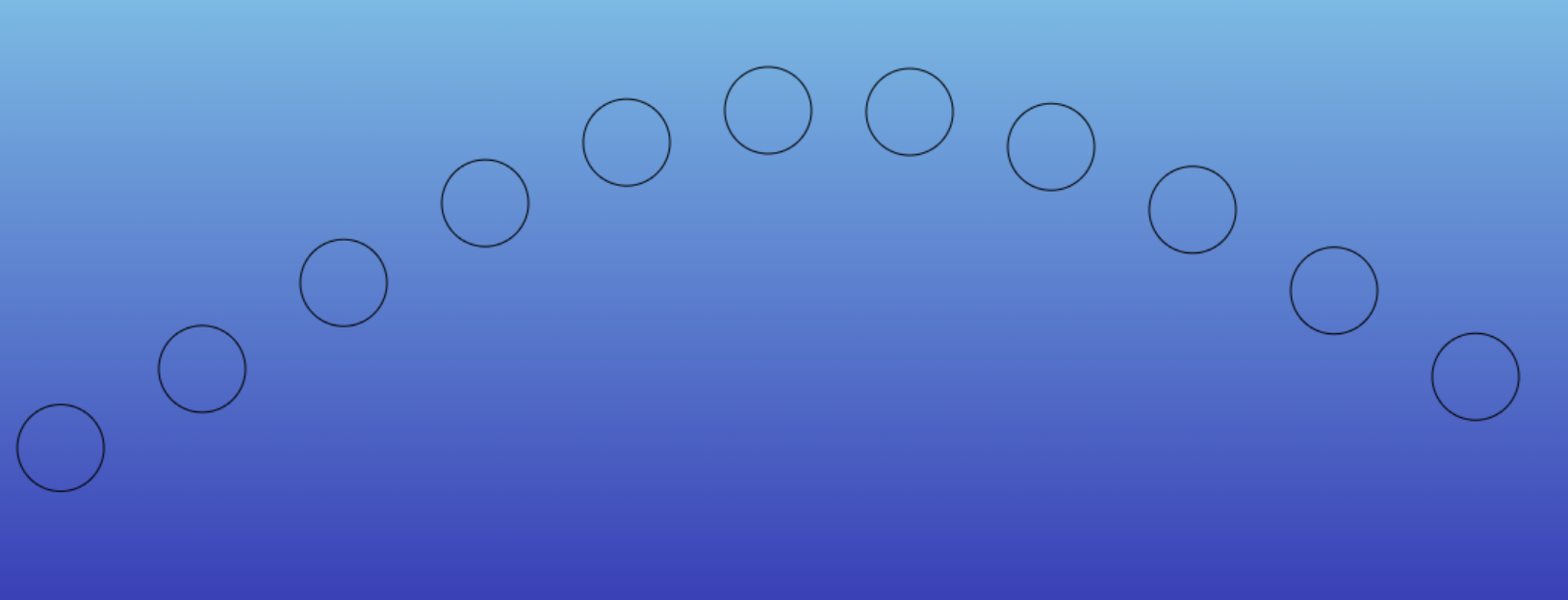
Every dragon needs a skeleton, and ours is no different. To capture the flowing, free spirit of the dragon, I decided to have its body follow the shape of a sine wave. This gives it an unearthly smoothness and makes the whole animation loop seamlessly. The basic design of the dragon's skeleton is based around this sine wave. All the points can be represented by a location on this wave. You can see this in the above image, where the circles are following the path of the wave.
Main Body
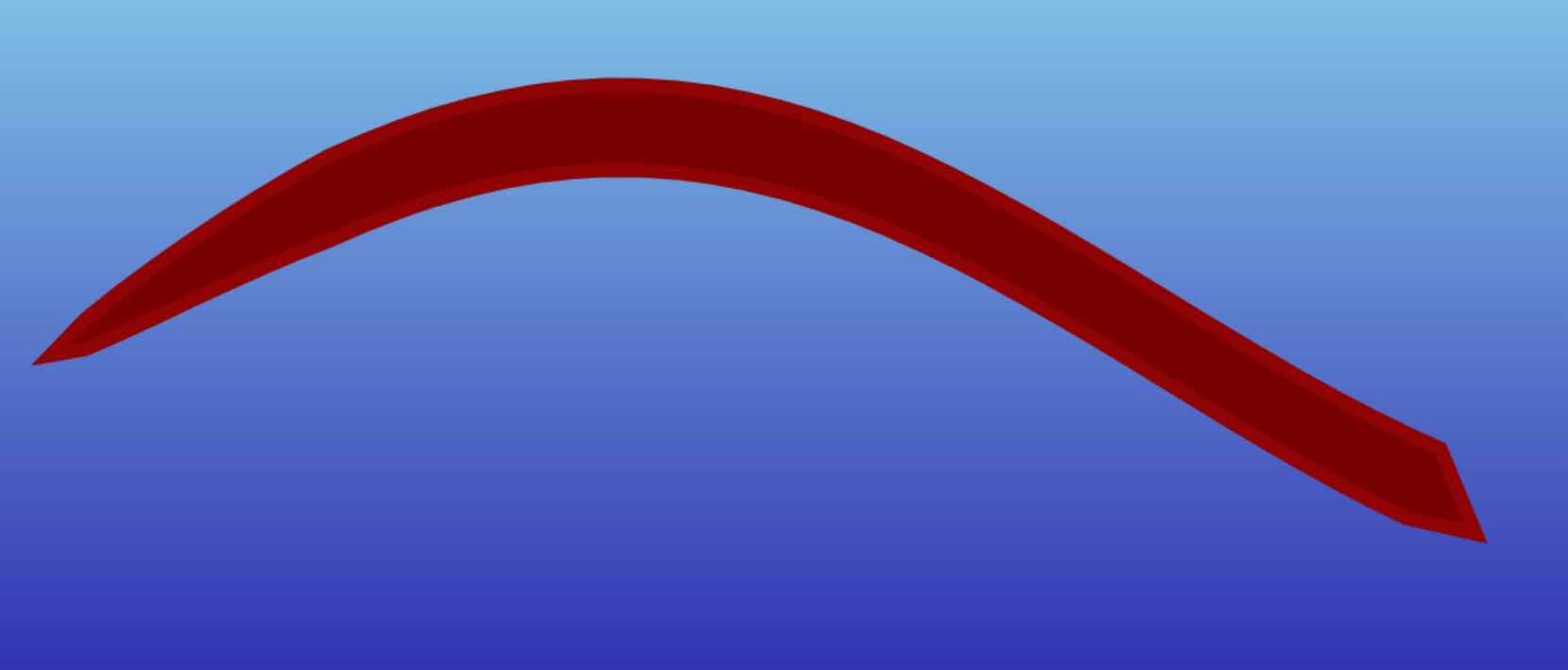
The main body of the dragon is created around our sine wave skeleton. By drawing lines between each point on the wave and offsetting them slightly, we get the dragon's main body and tail. The dragon is actually a bunch of line segments strung together, but they are so close together it appears seamless.
Scales
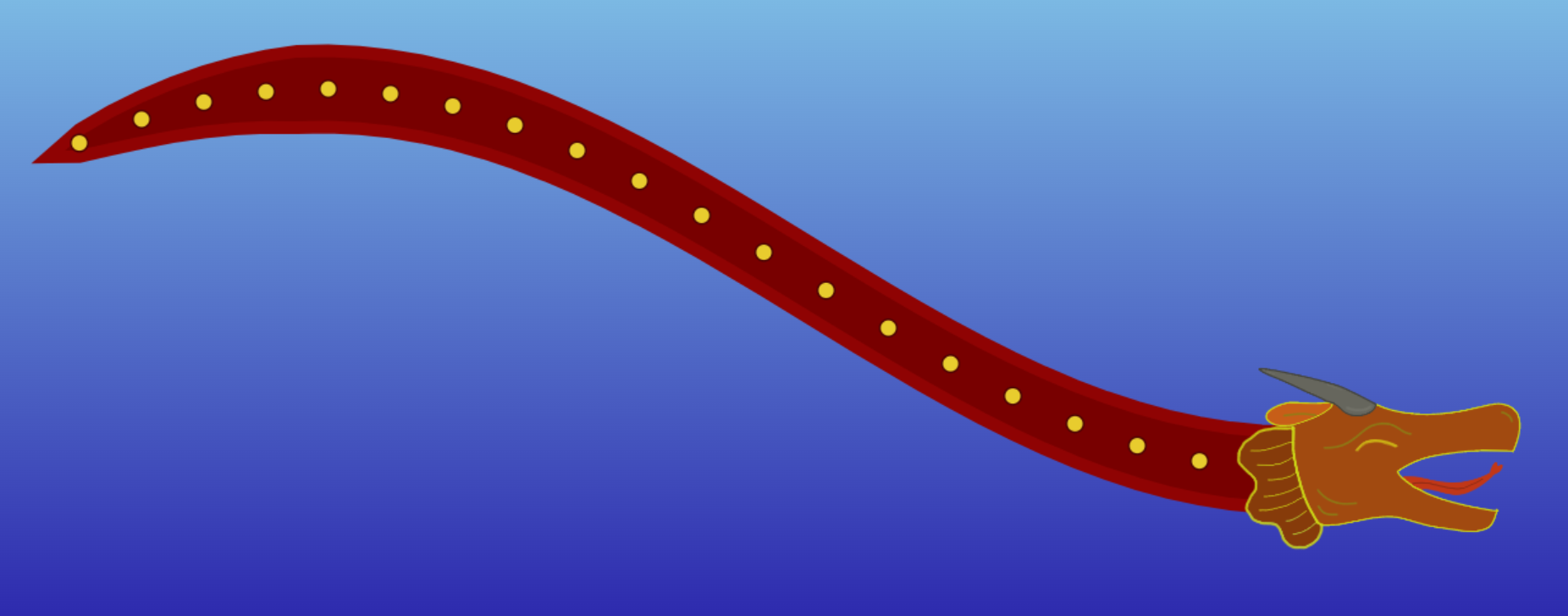
To give the dragon a little more detail, we will now add some scales. These follow the sine wave, just like the rest of the dragon, but they have no offset and therefore stay right in the middle. The scales are colored yellow to slightly contrast the red, but you can play around with the color if you like.
The Head
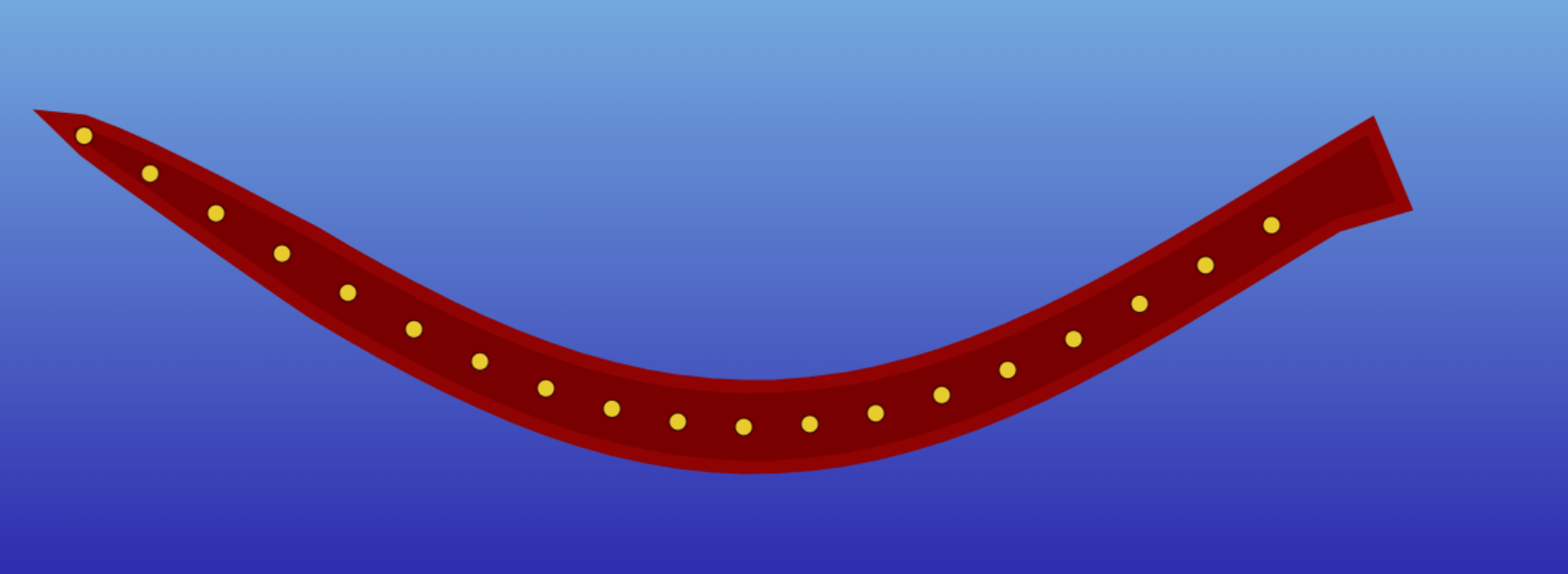
Now for the last part of the dragon - the head. Rather than trying to create the head using only Javascript (which would be a nightmare), I opted to create it using a paint app. Most computers come with one automatically installed, but you can also find some great online ones (which is where I created the head). If you don't want to make your own, you can just use the one I have here.
Clouds

As a finishing touch to really add to the atmosphere of the animation, let's add some clouds. My clouds are made with a single circular gradient (stored as an image and created in a paint app) that is randomly placed somewhere in a defined area. The whole area (and the points inside it) move across the screen, giving the idea that the dragon is flying. The clouds are semi-transparent, so they can be place in front of the dragon or behind it, whichever you want. All the cloud settings are adjustable in my code if you want to play around with them there.
Enjoy Your Animation!
If you've followed along and managed to create your own flying dragon, congratulations! If not, the code is available below. As mentioned before, you can control the cloud settings, but also the dragon's skeleton settings (things like wave speed and length). I thought this animation turned out pretty well for being coded in Javascript, and I felt like it was a good challenge. A lot of ideas had to be tinkered with and scrapped before this animation came into being, so I hope you enjoy it. Perhaps I'll make more Javascript animations, but until then, have a great rest of your day!
Downloads
Code and Files
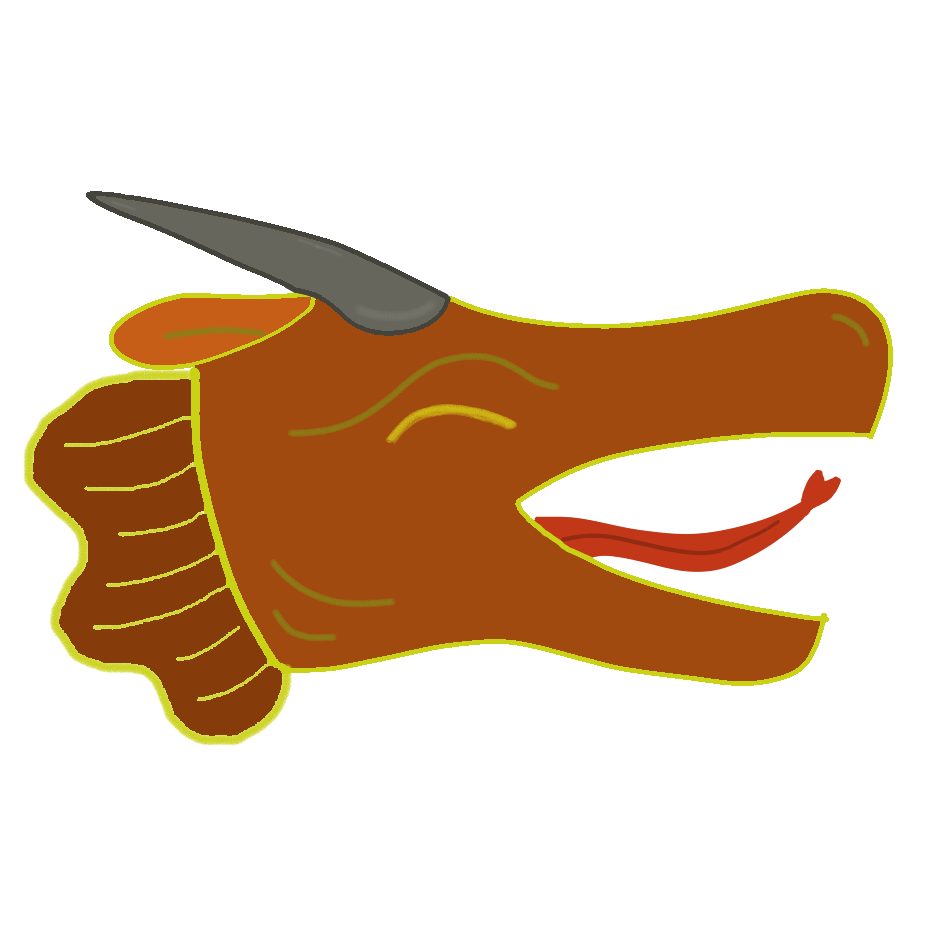
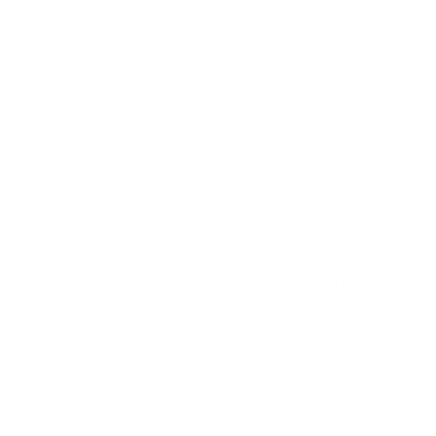
HTML
Javascript